Who don’t know what jQuery is and how it can help in developing a web project. Based on the latest statistics, jQuery is used on ~60% of the Quantcast Top 100k websites. We use it constantly and sometimes we tend to think it’s almost magic, that jQuery is capable to understand what we want to achieve and optimize all for us. There is no need to say this is wrong. You need to be aware of what jQuery does and does not for you and optimize as much as you can to improve your website’s performance.
In this article, extracted from my book Instant jQuery Selectors, you’ll learn some useful tips and tricks to improve the performance of your website by simply selecting elements in the right way.
Please note that, to be a complete post, it has some minor adjustments compared to the original recipe titled How to have efficient selectors (Become an expert).
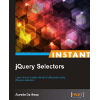
This article is extracted from my book Instant jQuery Selectors. Consider buying it on the Packt Publishing website or on Amazon.
Instant jQuery Selectors is a practical guide that will teach you how to use jQuery’s selectors efficiently in order to easily select the elements of your pages to operate upon with jQuery’s methods. You will go through the most common problems that you could face while developing your project and will learn how to solve them with the help of focused examples that Instant jQuery Selectors has to offer.
How to do it…
To boost the performances, keep in mind the following points.
1. Avoid the Universal Selector
Don’t use the Universal selector (explicitly or implicitly). Never! Seriously!
2. Rely on Native Functions
The best selectors are the Id, Class and Element selectors, because under the hood jQuery uses native JavaScript functions.
3. Id selector is the Best
The best performances are achieved using the ID selector.
4. Don’t Overqualify the Id Selector
Never prepend a tag name before an id, it’ll slow down the selector. For example, don’t turn
$('#content')
into
$('div#content')
jQuery starts parsing the selector using a regular expression. If it discloses that the selectors is an id, because it starts with a sharp (#
), behind the scenes jQuery will use the JavaScript native getElementById()
method, that is very fast.
5. Start with the Id Selector when Possible
If your selection needs more than one selector, start with the Id selector if possible. For example:
$('#content p.border')
6. Don’t Forget to Use the context Parameter
Remember to use the context
parameter or the find()
method when possible. For example, turn
$('#content p.border')
into
$('p.border', '#content')
or even better into
$('#content').find('p.border')
The reason is that the jQuery constructor will do nothing but convert $('p.border', '#content')
into $('#content').find('p.border')
. If you look at the source code, you’ll find these lines:
// HANDLE: $(expr, context) // (which is just equivalent to: $(context).find(expr) } else { return this.constructor( context ).find( selector ); }
7. Be More Specific on the Right Side
jQuery’s selectors engine, called Sizzle, parses selectors from right to left. Therefore, to speed up your selection, be more specific on the right part and less on the left. For example, turn
$('div.wrapper .border')
into
$('.wrapper p.border')
8. Don’t be too Specific
Don’t be too specific if the same set can be selected with less selectors. For example:
$('#content p.border')
is better than
$('#content div.wrapper div p.border')
9. Don’t be too Generic when Using Filters
If you need to use a filter, it’s always better to narrow down the set of elements and then use the filter. For example, turn
$(':enabled')
into
$('#formId').find(':enabled')
or even better
$('#formId').find('input:enabled')
10. Use filter() instead of jQuery’s Selectors Extension
Filters such as, :button
, :checkbox
, :visible
, :input
, and others are a jQuery extension and not part of the CSS specification, so they can’t take advantage of the performance provided by querySelectorAll()
. To have better performance, it’s better to first select elements using a pure CSS selector and then filter using filter()
(that is, .filter(':input')
).
11. Use the Attribute Selector instead of jQuery’s Selectors Extension
Filters such as, :image
, :text
, :password
, :reset
and others are a jQuery extension and not part of the CSS specification, so they can’t take advantage of the performance provided by querySelectorAll()
. To have better performance, it’s better to select using the attribute selector. For example, turn
$(':reset')
into
$('[type=reset]')
12. Be Careful of Not Equal Attribute Selector
To improve the performance of the Not Equal attribute selector in browsers that support querySelectorAll()
, use the not()
method. For example, turn
$('.border [placeholder!=Age]')
into
$('.border').not('[placeholder=Age]')
13. Be Careful of the :lt() Filter
To improve the performance of :lt()
filter in modern browsers, use the slice()
method. For example, turn
$('.border:lt(2)')
into
$('.border').slice(0, 3)
14. Be Careful of the :gt() Filter
To improve the performance of the :gt()
filter in modern browsers, use the slice()
method. For example, turn
$('.border:gt(2)')
into
$('.border').slice(3)
15. Be Careful of the :has() Filter
To improve the performance of the :has()
filter in modern browsers, use the has()
method. For example, turn
$('div:has(p.border)')
into
$('div').has('p.border')
Conclusions
In this article I explained how you can improve the performance of your web pages simply paying attention to how you select elements using jQuery. Keep in mind that these tips aren’t magic, so just applying them your page that currently loads in 10 seconds won’t turn into one that loads in 2 seconds. However, if your operations on the elements you retrieve are complex enough, just tweaking your selectors you can see some performance improvements.
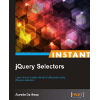
If you liked this article, consider buying my book Instant jQuery Selectors on the Packt Publishing website or on Amazon.
Instant jQuery Selectors is for web developers who want to delve into jQuery from its very starting point: selectors. Even if you’re already familiar with the framework and its selectors, you could find several tips and tricks that you aren’t aware of, especially about performance and how jQuery acts behind the scenes.